CS162 PintOS
Jan 1, 2024
·
2 min read
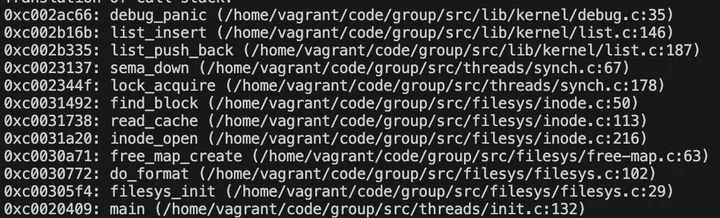
I implemented part of an operating system called Pintos.
For this project in UC Berkeley’s CS162, I implemented part of an operating system called Pintos. The OS supports multi-threading, virtual memory, FFS file system, and user programs. The OS is written in C and x86 assembly.
The parts I implemented (with my team) are:
- User program setup and syscall handling
- Parsing user program arguments and passing them onto the user stack
- Setting up stack and heap for the user program
- Initializing PCB and passing load information to the PCB
- Sleeping the parent process, passing info to the parent process waiting on the child process to load, and waking up the parent process
- Implementing
wait
,exec
,exit
,sbrk
- Implementing FPU context initialization, saving, and restoring
- File System
- Implemented fs cache blocks using LRU eviction policy, free map, extensible inode, directory structures, and file structure
- Implemented file system calls
create
,remove
,open
,close
,read
,write
,seek
,tell
,filesize
,mkdir
,readdir
,isdir
,inumber
,chdir
- Multithreading
- Implemented thread scheduling using priority scheduling
- Implemented thread
alarm
system call - Implemented user level threads
- Designing and implementing thread control block
- Designing and implementing kernel level user thread trapping (to allow user threads to get terminated by the kernel)
- Implementing pthread library functions
pthread_create
,pthread_exit
,pthread_join
,pthread_mutex_init
,pthread_mutex_lock
,pthread_mutex_unlock
,pthread_sema_init
,pthread_sema_up
,pthread_sema_down
.